Video Demo:
Navigation Drawer is used to display the sliding panel of android application. It is the most important part of the each application. Navigation drawer is not always in the open state. To see the Navigation drawer user need to click on menu option or slide to left. In this tutorial I am creating an application with Navigation drawer using kotlin Download source code from below.
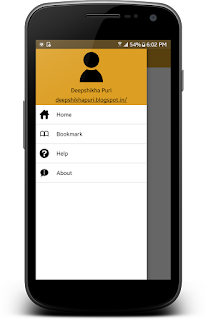
activity_main.xml:
DOWNLOAD SOURCE CODE FROM HERE
Navigation Drawer is used to display the sliding panel of android application. It is the most important part of the each application. Navigation drawer is not always in the open state. To see the Navigation drawer user need to click on menu option or slide to left. In this tutorial I am creating an application with Navigation drawer using kotlin Download source code from below.
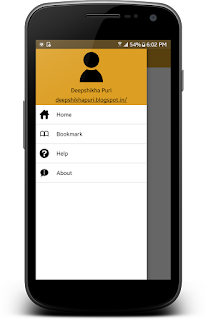
activity_main.xml:
<?xml version="1.0" encoding="utf-8"?>
<android.support.v4.widget.DrawerLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/drawer_layout"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#ffffff"
android:fitsSystemWindows="true"
tools:openDrawer="start">
<RelativeLayout
android:id="@+id/rl_main"
android:layout_width="match_parent"
android:layout_height="match_parent">
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="50dp"
android:background="@color/colorPrimary">
<RelativeLayout
android:id="@+id/rl_menu"
android:layout_width="40dp"
android:layout_height="match_parent">
<ImageView
android:layout_width="25dp"
android:layout_height="25dp"
android:layout_centerVertical="true"
android:layout_marginLeft="10dp"
android:src="@drawable/menu4" />
</RelativeLayout>
<TextView
android:id="@+id/tv_title"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerInParent="true"
android:text="Navigation drawer"
android:textColor="#000000"
android:textSize="15dp"
android:textStyle="bold" />
</RelativeLayout>
<TextView
android:id="@+id/tv_home"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerInParent="true"
android:textColor="#dc9e23"
android:textSize="25dp"
android:textStyle="bold" />
</RelativeLayout>
<!--Menu Options-->
<LinearLayout
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:layout_gravity="start"
android:background="#ffffff"
android:orientation="vertical">
<LinearLayout
android:id="@+id/rl_header"
android:layout_width="match_parent"
android:layout_height="150dp"
android:background="#dc9e23"
android:orientation="vertical">
<ImageView
android:layout_width="100dp"
android:layout_height="100dp"
android:layout_gravity="center"
android:src="@drawable/profile2" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:autoLink="web"
android:paddingBottom="5dp"
android:text="Deepshikha Puri"
android:textColor="#000000"
android:textSize="15dp" />
<TextView
android:id="@+id/tv_link"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:autoLink="web"
android:paddingBottom="5dp"
android:text="deepshikhapuri.blogspot.in/"
android:textColor="#000000"
android:textSize="15dp"
android:visibility="visible" />
</LinearLayout>
<LinearLayout
android:id="@+id/ll_home"
android:layout_width="match_parent"
android:layout_height="50dp"
android:orientation="horizontal">
<ImageView
android:layout_width="25dp"
android:layout_height="25dp"
android:layout_gravity="center_vertical"
android:layout_marginLeft="10dp"
android:src="@drawable/home" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:layout_marginLeft="20dp"
android:text="Home"
android:textColor="#000000"
android:textSize="15dp" />
</LinearLayout>
<View
android:layout_width="match_parent"
android:layout_height="2dp"
android:background="#eaeaea"></View>
<LinearLayout
android:id="@+id/ll_bookmark"
android:layout_width="match_parent"
android:layout_height="50dp"
android:orientation="horizontal">
<ImageView
android:layout_width="25dp"
android:layout_height="25dp"
android:layout_gravity="center_vertical"
android:layout_marginLeft="10dp"
android:src="@drawable/bookmark" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:layout_marginLeft="20dp"
android:text="Bookmark"
android:textColor="#000000"
android:textSize="15dp" />
</LinearLayout>
<View
android:layout_width="match_parent"
android:layout_height="2dp"
android:background="#eaeaea"></View>
<LinearLayout
android:id="@+id/ll_help"
android:layout_width="match_parent"
android:layout_height="50dp"
android:orientation="horizontal">
<ImageView
android:layout_width="25dp"
android:layout_height="25dp"
android:layout_gravity="center_vertical"
android:layout_marginLeft="10dp"
android:src="@drawable/help" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:layout_marginLeft="20dp"
android:text="Help"
android:textColor="#000000"
android:textSize="15dp" />
</LinearLayout>
<View
android:layout_width="match_parent"
android:layout_height="2dp"
android:background="#eaeaea"></View>
<LinearLayout
android:id="@+id/ll_about"
android:layout_width="match_parent"
android:layout_height="50dp"
android:orientation="horizontal">
<ImageView
android:layout_width="25dp"
android:layout_height="25dp"
android:layout_gravity="center_vertical"
android:layout_marginLeft="10dp"
android:src="@drawable/about" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:layout_marginLeft="20dp"
android:text="About"
android:textColor="#000000"
android:textSize="15dp" />
</LinearLayout>
<View
android:layout_width="match_parent"
android:layout_height="2dp"
android:background="#eaeaea"></View>
</LinearLayout>
</android.support.v4.widget.DrawerLayout>
MainActivity.kt:package navigationdrawer.deepshikha.com.navigationdrawerkotlin
import android.graphics.Color
import android.os.Bundle
import android.support.v4.view.GravityCompat
import android.support.v7.app.AppCompatActivity
import android.text.method.LinkMovementMethod
import android.text.util.Linkify
import android.view.View
import kotlinx.android.synthetic.main.activity_main.*
import android.content.Intent
import android.net.Uri
class MainActivity : AppCompatActivity(), View.OnClickListener {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
init()
}
private fun init() {
tv_home.setText("Home Tab")
tv_title.setText("Home")
ll_about.setOnClickListener(this)
ll_home.setOnClickListener(this)
ll_bookmark.setOnClickListener(this)
ll_help.setOnClickListener(this)
rl_menu.setOnClickListener(this)
rl_header.setOnClickListener(this)
tv_link.setLinkTextColor(Color.parseColor("#000000"));
Linkify.addLinks(tv_link, Linkify.ALL)
}
override fun onBackPressed() {
if (drawer_layout.isDrawerOpen(GravityCompat.START)) {
drawer_layout.closeDrawer(GravityCompat.START)
} else {
super.onBackPressed()
}
}
override fun onClick(p0: View?) {
when (p0?.id) {
R.id.ll_home -> {
drawer_layout.closeDrawer(GravityCompat.START)
tv_home.setText("Home Tab")
tv_title.setText("Home")
}
R.id.ll_about -> {
val browserIntent = Intent(Intent.ACTION_VIEW, Uri.parse("http://deepshikhapuri.blogspot.in/"))
startActivity(browserIntent)
}
R.id.ll_help -> {
drawer_layout.closeDrawer(GravityCompat.START)
tv_home.setText("Help Tab")
tv_title.setText("Help")
}
R.id.ll_bookmark -> {
drawer_layout.closeDrawer(GravityCompat.START)
tv_home.setText("Bookmark Tab")
tv_title.setText("Bookmark")
}
R.id.rl_header -> {
}
R.id.rl_menu -> {
if (drawer_layout.isDrawerOpen(GravityCompat.START)) {
drawer_layout.closeDrawer(GravityCompat.START)
} else {
drawer_layout.openDrawer(GravityCompat.START)
}
}
}
}
}
DOWNLOAD SOURCE CODE FROM HERE